Node.js is celebrated for its efficiency and scalability, but what makes it truly shine is its non-blocking, asynchronous architecture. At the heart of this system lies the event loop, a fundamental concept that powers the magic of asynchronous programming. Let’s break it down into simple terms.
What is the Event Loop?
The event loop is the core mechanism that enables Node.js to handle multiple tasks simultaneously without threads. It operates on a single-threaded model but uses an underlying C++ thread pool for heavy lifting.
When your Node.js application starts, the event loop begins its cycle. Tasks like reading files or querying databases are sent to the thread pool, allowing the main thread to continue running without waiting for these operations to complete. This ensures your app remains fast and responsive.
How Async Programming Works in Node.js
Asynchronous programming is a method of running code without waiting for operations to finish. In Node.js, it’s implemented using:
- Callbacks: The traditional way, where a function is passed to handle the result once a task is complete.
- Promises: A more modern approach that offers a cleaner syntax and better error handling.
- Async/Await: Built on promises, it provides a synchronous-like way to write asynchronous code, making it easier to read and maintain.
Here’s an example using async/await:
const fs = require('fs').promises;
async function readFile() {
try {
const data = await fs.readFile('example.txt', 'utf-8');
console.log(data);
} catch (error) {
console.error('Error reading file:', error);
}
}
readFile();
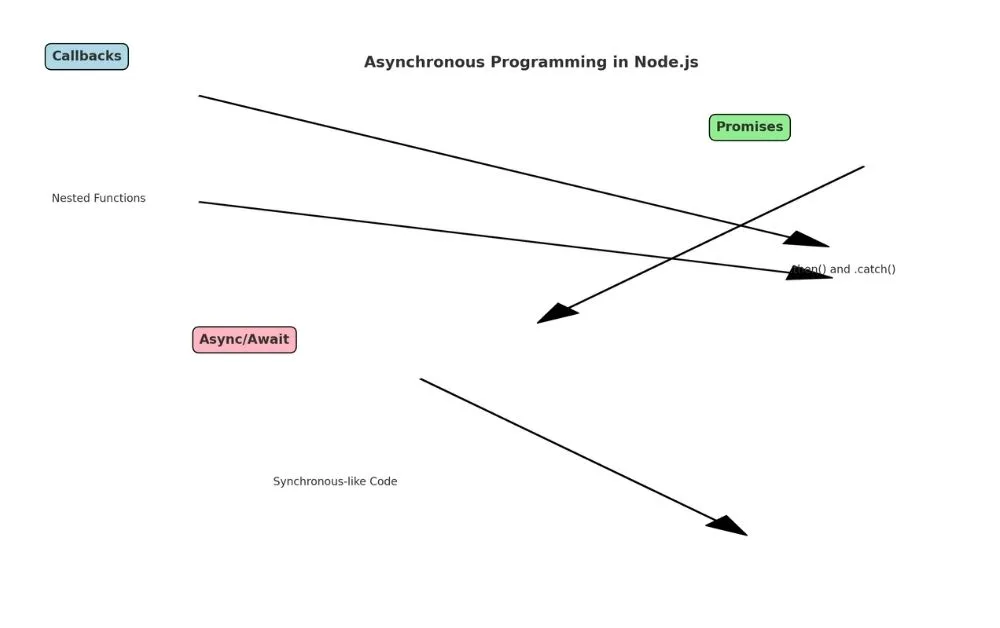
Why Does the Event Loop Matter?
Understanding the event loop helps you write efficient code. For instance, blocking operations on the main thread (like large computations) can freeze your application. Instead, offload such tasks to worker threads or use libraries designed for heavy processing.
The event loop also helps handle timers (setTimeout, setInterval) and I/O tasks efficiently, ensuring smooth application performance.
Common Challenges with Async Programming
While the event loop makes Node.js fast, it also introduces challenges like:
- Callback Hell: A pyramid of nested callbacks that’s hard to read and debug. Promises and async/await help mitigate this.
- Unhandled Rejections: Forgetting to handle promise rejections can lead to crashes. Always use
.catch()
or a try-catch block.
Conclusion: Mastering the Event Loop
The event loop is the unsung hero of Node.js, enabling non-blocking I/O and efficient multitasking. Asynchronous programming, while challenging at first, becomes second nature with practice. Understanding how the event loop works will empower you to write performant and reliable applications in Node.js.
Ready to dive deeper? Start experimenting with async tasks today and see the event loop in action!
Asynchronous JavaScript: Promises, Async/Await, and Callbacks