Integrating AI functionalities into a React application
can significantly boost its capabilities. Preparing the development environment is the initial step, ensuring all necessary tools and packages are in place. From there, you can create an interface that harnesses the power of OpenAI's APIs
to provide dynamic responses to user inputs.
Setting Up the Development Environment
To begin integrating AI functionalities into a React application
, prepare your development environment:
- Confirm Node.js and npm are installed. If not, download and install them from the Node.js website.
- Create a new React application:
npx create-react-app my-app
cd my-app - Set up the backend for AI API calls:
mkdir api
cd api
npm install openai - Install Azure Functions Core Tools for local development and testing.
- Set up your OpenAI API key in
local.settings.json
:
{
"IsEncrypted": false,
"Values": {
"FUNCTIONS_WORKER_RUNTIME": "node",
"OPENAI_API_KEY": "your_api_key_here"
}
} - Create an endpoint in
/api/index.js
to handle requests:
The code for the endpoint includes setting up the OpenAI configuration
, creating functions to handle text and image generation, and returning the response to the client.
Finally, create the front-end interface in /src/App.js
. This interface will include a textarea for user input, a submit button, and areas to display the AI-generated text and image responses.
Integrating OpenAI APIs with React
To incorporate OpenAI's GPT
and DALL-E APIs
into your React application, you’ll need to make updates to both the backend and frontend code:
1. Update /api/index.js
:
This file will handle the API requests to OpenAI, including both text generation (using GPT) and image generation (using DALL-E). The code includes error handling and returns the generated text and image URL to the frontend.
2. Update /src/App.js
:
The frontend React component will manage the state of the application, including:
- User input
- Generated response text
- Generated image URL
- Loading state
- Error messages
The handleSubmit
function sends the user’s input to the backend, handles the response, and updates the component’s state accordingly.
Key Features:
- Real-time user input handling
- Asynchronous API calls
- Dynamic UI updates based on API responses
- Error handling and user feedback
This setup allows your React application to leverage the power of OpenAI's GPT
and DALL-E
capabilities, generating dream analysis and visualizations based on user input.
Building Real-Time Conversational AI
To implement real-time conversational AI in your React application
, you’ll need to modify your existing setup to maintain a conversation history and provide contextual responses. Here’s how to achieve this:
1. Update /src/App.js
:
The main React component
needs to be updated to handle a conversation flow rather than single-message interactions. Key changes include:
- Maintaining a
messages
state array to store the conversation history - Updating the UI to display the entire conversation
- Modifying the
handleSubmit
function to append new messages and make API calls
2. Create /api/chat.js
:
This new backend file will handle the chat-specific API calls to OpenAI. It takes the entire conversation history as input and returns the AI’s response based on the context of the conversation.
3. Update /api/index.js
:
Modify the existing backend file to incorporate the new chat functionality alongside the dream analysis features. This allows for a seamless integration of both capabilities in your application.
“By maintaining conversation context, AI can provide more relevant and engaging responses, creating a more natural and interactive user experience.”
With these modifications, your React application now incorporates real-time conversational AI, maintaining context across messages and providing relevant responses to user inputs. This enhanced interactivity can significantly improve user engagement and the overall functionality of your AI-powered application.
Dynamic Image Generation with OpenAI
To add dynamic image generation using OpenAI’s DALL-E API, update your backend in /api/index.js
:
const { Configuration, OpenAIApi } = require('openai');
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY
});
const openai = new OpenAIApi(configuration);
module.exports = async function (context, req) {
const inputText = req.body.inputText;
try {
const imageResponse = await openai.createImage({
prompt: inputText,
n: 1,
size: "512x512"
});
const generatedImage = imageResponse.data.data[0].url;
context.res = {
status: 200,
body: {
text: responseText?.data?.choices[0]?.message?.content || 'Generated text description will be here',
image: generatedImage,
response: chatResponse?.data?.choices[0]?.message?.content || 'Conversational AI response will be here'
}
};
} catch (error) {
context.res = {
status: 500,
body: { error: 'Error generating response from OpenAI' }
};
}
};
Modify the front-end interface in /src/App.js
:
import { useState } from 'react';
import './App.css';
function App() {
const [inputText, setInputText] = useState('');
const [responseText, setResponseText] = useState('');
const [imageUrl, setImageUrl] = useState('');
const [loading, setLoading] = useState(false);
const [error, setError] = useState('');
const handleSubmit = async () => {
if (!inputText.trim()) {
setError('Please enter a description or message.');
return;
}
setLoading(true);
setError('');
try {
const response = await fetch('/api/index', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ inputText })
});
const data = await response.json();
setResponseText(data.text);
setImageUrl(data.image);
} catch (e) {
setError('Failed to get response from server.');
} finally {
setLoading(false);
}
};
return (
setInputText(e.target.value)}
placeholder="Describe your dream or input text here"
/>
{error && <p className="error">{error}</p>}
<button onClick={handleSubmit} disabled={loading}>
{loading ? 'Analyzing...' : 'Generate'}
</button>
<div>
{responseText && <p>{responseText}</p>}
{imageUrl && <p>Generated image URL: {imageUrl}</p>}
</div>
</div>
);
}
export default App;
</code>
<p>This setup allows users to input text descriptions and receive both generated text and visual representations, combining OpenAI's GPT-3 and DALL-E capabilities. Here's a breakdown of the key features:</p>
<ul>
<li><b>Dynamic Image Generation:</b> Utilizes DALL-E API to create images based on user input.</li>
<li><b>Text Generation:</b> Incorporates GPT-3 for generating textual responses.</li>
<li><b>Error Handling:</b> Implements robust error checking and user feedback.</li>
<li><b>Responsive UI:</b> Provides a simple, user-friendly interface for input and output display.</li>
</ul>
<p>The combination of these technologies opens up exciting possibilities for <i>creative applications</i>, such as:</p>
<ol>
<li>Visual storytelling tools</li>
<li>Idea visualization for brainstorming sessions</li>
<li>Educational aids for complex concepts</li>
<li>Personalized art generation</li>
</ol>
<p>It's important to note that while this implementation is powerful, it should be used responsibly. Always consider ethical implications and potential biases in AI-generated content<sup>1</sup>.</p>
</section>
<section id="references">
<ol>
<li>Bender EM, Gebru T, McMillan-Major A, Shmitchell S. On the Dangers of Stochastic Parrots: Can Language Models Be Too Big? In: Proceedings of the 2021 ACM Conference on Fairness, Accountability, and Transparency. ACM; 2021:610-623.</li>
</ol>
</section><section id="section-5">
<h3>Enhancing User Experience with QuickBlox AI Libraries</h3>
<p>QuickBlox AI libraries can significantly improve user interactions and communication in web applications. This section covers how to install, configure, and implement <b>qb-ai-answer-assistant</b>, <b>qb-ai-translate</b>, and <b>qb-ai-rephrase</b>.</p>
<p>First, ensure you have Node.js and npm installed. Then, add the QuickBlox AI libraries to your project:</p>
<code>
npm install qb-ai-answer-assistant qb-ai-translate qb-ai-rephrase --save
</code>
<h5>Setting Up QuickBlox AI Answer Assistant</h5>
<p>Create <code>assistController.js</code> in your backend directory:</p>
<code>
const QBAIAnswerAssistant = require('qb-ai-answer-assistant').QBAIAnswerAssistant;
const history = [
{ role: "user", content: "Good afternoon. Do you like football?" },
{ role: "assistant", content: "Hello. I've been playing football all my life." },
{ role: "user", content: "Can you please explain the rules of playing football?"}
];
const textToAssist = "Can you please explain the rules of playing football?";
module.exports.showFormAssist = async function (req, res) {
try {
const settings = QBAIAnswerAssistant.createDefaultAIAnswerAssistantSettings();
settings.apiKey = process.env.QB_API_KEY;
settings.model = 'gpt-3.5-turbo';
settings.maxTokens = 3584;
const result = await QBAIAnswerAssistant.createAnswer(req.body.text, history, settings);
res.render('assist', {
title: 'AI Assist',
isAssist: true,
history: JSON.stringify(history, null, 4),
textToAssist,
result
});
} catch (e) {
res.render('assist', {
title: 'AI Assist',
isAssist: true,
history: JSON.stringify(history, null, 4),
textToAssist,
result: 'Error assist'
});
}
};
</code>
<h5>Implementing QuickBlox AI Translate</h5>
<p>Update <code>translateController.js</code>:</p>
<code>
const QBAITranslate = require('qb-ai-translate').QBAITranslate;
const history = [
{ role: "user", content: "Good afternoon. Do you like football?" },
{ role: "assistant", content: "Hello. I've been playing football all my life." }
];
module.exports.aiCreateTranslate = async function (req, res) {
try {
const settings = QBAITranslate.createDefaultAITranslateSettings();
settings.apiKey = process.env.QB_API_KEY;
settings.model = 'gpt-3.5-turbo';
settings.language = req.body.language || 'English';
settings.maxTokens = 3584;
const result = await QBAITranslate.translate(req.body.text, history, settings);
res.render('translate', {
title: 'AI Translate',
isTranslate: true,
history: JSON.stringify(history, null, 4),
textToTranslate: req.body.text,
result
});
} catch (e) {
res.render('translate', {
title: 'AI Translate',
isTranslate: true,
history: JSON.stringify(history, null, 4),
textToTranslate: req.body.text,
result: 'Error translate'
});
}
}
</code>
<h5>Configuring QuickBlox AI Rephrase</h5>
<p>Create <code>rephraseController.js</code>:</p>
<code>
const QBAIRephrase = require('qb-ai-rephrase').QBAIRephrase;
const history = [
{ role: "user", content: "Good afternoon. Do you like football?" },
{ role: "assistant", content: "Hello. I've been playing football all my life." },
{ role: "user", content: "Can you please explain the rules of playing football?"}
];
const tones = [
{ name: 'Professional Tone', iconEmoji: '👔' },
{ name: 'Friendly Tone', iconEmoji: '🤝' },
{ name: 'Encouraging Tone', iconEmoji: '💪' },
{ name: 'Empathetic Tone', iconEmoji: '🤲' },
{ name: 'Neutral Tone', iconEmoji: '😐' },
{ name: 'Assertive Tone', iconEmoji: '🔨' },
{ name: 'Instructive Tone', iconEmoji: '📖' },
{ name: 'Persuasive Tone', iconEmoji: '☝️' },
{ name: 'Sarcastic/Ironic Tone', iconEmoji: '😏' },
{ name: 'Poetic Tone', iconEmoji: '🎭' }
];
const getToneByName = (toneName) => {
return tones.find(tone => tone.name === toneName) || tones[0];
}
module.exports.aiCreateRephrase = async function (req, res) {
try {
const settings = QBAIRephrase.createDefaultAIRephraseSettings();
settings.apiKey = process.env.QB_API_KEY;
settings.model = 'gpt-3.5-turbo';
settings.tone = getToneByName(req.body.tone);
settings.maxTokens = 3584;
const result = await QBAIRephrase.rephrase(req.body.text, history, settings);
res.render('rephrase', {
title: 'AI Rephrase',
isRephrase: true,
history: JSON.stringify(history, null, 4),
textToRephrase: req.body.text,
tones,
result
});
} catch (e) {
res.render('rephrase', {
title: 'AI Rephrase',
isRephrase: true,
history: JSON.stringify(history, null, 4),
textToRephrase: req.body.text,
tones,
result: 'Error rephrase'
});
}
}
</code>
<h5>Integrating React Front-End</h5>
<p>Update <code>/src/App.js</code>:</p>
<code>
import { useState } from 'react';
import './App.css';
function App() {
const [inputText, setInputText] = useState('');
const [responseText, setResponseText] = useState('');
const [translatedText, setTranslatedText] = useState('');
const [rephrasedText, setRephrasedText] = useState('');
const [loading, setLoading] = useState(false);
const [error, setError] = useState('');
const [mode, setMode] = useState('assist');
const handleSubmit = async () => {
setLoading(true);
setError('');
try {
const endpoint = mode === 'assist' ? '/api/assist' : mode === 'translate' ? '/api/translate' : '/api/rephrase';
const response = await fetch(endpoint, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ text: inputText })
});
const data = await response.json();
if (mode === 'assist') setResponseText(data.result);
if (mode === 'translate') setTranslatedText(data.result);
if (mode === 'rephrase') setRephrasedText(data.result);
} catch (e) {
setError('Failed to get response from server.');
} finally {
setLoading(false);
}
};
return (
<div className="App">
<textarea
value={inputText}
onChange={(e) => setInputText(e.target.value)}
placeholder="Enter your text here"
/>
{error && <p className="error">{error}</p>}
<button onClick={() => setMode('assist')}>AI Assist</button>
<button onClick={() => setMode('translate')}>AI Translate</button>
<button onClick={() => setMode('rephrase')}>AI Rephrase</button>
<button onClick={handleSubmit} disabled={loading}>
{loading ? 'Processing...' : 'Submit'}
</button>
<div>
{mode === 'assist' && <p>{responseText}</p>}
{mode === 'translate' && <p>{translatedText}</p>}
{mode === 'rephrase' && <p>{rephrasedText}</p>}
</div>
</div>
);
}
export default App;
</code>
<p>These QuickBlox AI libraries offer <i>powerful AI capabilities</i> that can significantly improve user engagement in your web application. By integrating these tools, you can create a more <b>interactive</b> and <b>personalized</b> experience for your users.</p>
<h5>Key Benefits of QuickBlox AI Libraries</h5>
<ul>
<li><b>Enhanced User Interaction:</b> The AI Answer Assistant can provide instant, context-aware responses to user queries.</li>
<li><b>Multilingual Support:</b> AI Translate breaks down language barriers, making your application accessible to a global audience.</li>
<li><b>Improved Communication:</b> AI Rephrase helps users convey their messages more effectively by adapting to different tones and styles.</li>
<li><b>Scalability:</b> These libraries can handle a large number of requests simultaneously, ensuring smooth performance even with high user traffic.</li>
</ul>
<p>By leveraging these AI-powered tools, you can create a more engaging, efficient, and user-friendly web application that stands out in today's competitive digital landscape.</p>
</section>
<section id="references">
<ol>
<li>QuickBlox. QuickBlox AI Libraries Documentation. QuickBlox; 2023.</li>
<li>OpenAI. GPT-3.5 Turbo Model Overview. OpenAI; 2023.</li>
<li>React. React Documentation. Facebook Open Source; 2023.</li>
</ol>
</section><section id="section-6">
<h3>Advanced AI Features with Next.js</h3>
<p>To integrate advanced AI features into a Next.js application using OpenAI's APIs, follow these steps:</p>
<ol>
<li><b>Initialize your Next.js project:</b>
<code>
npx create-next-app@latest ai-nextjs-integration
cd ai-nextjs-integration
</code>
</li>
<li><b>Set up the backend to handle API calls to OpenAI:</b>
<ul>
<li>Install the OpenAI SDK:
<code>npm install openai</code>
</li>
<li>Create <code>message.js</code> in the <code>pages/api</code> directory for handling AI responses:</li>
</ul>
</li>
</ol>
<code>
// pages/api/message.js
import { Configuration, OpenAIApi } from 'openai';
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY
});
const openai = new OpenAIApi(configuration);
export default async function handler(req, res) {
const { messages } = req.body;
try {
const aiResponse = await openai.createChatCompletion({
model: "gpt-3.5-turbo",
messages: [
{ role: "system", content: "You are an intelligent chatbot." },
...messages
]
});
res.status(200).json({ response: aiResponse.data.choices[0].message.content });
} catch (error) {
res.status(500).json({ error: 'Error generating response from OpenAI' });
}
}
</code>
<p>Create a <code>.env.local</code> file in your project root to store your OpenAI API key:</p>
<code>
OPENAI_API_KEY=your_openai_api_key_here
</code>
<p><i>Set up the front-end to handle user interactions and display responses.</i> Modify <code>index.js</code> in the <code>pages</code> directory:</p>
<code>
// pages/index.js
import { useState } from 'react';
import Head from 'next/head';
import styles from '../styles/Home.module.css';
export default function Home() {
const [messages, setMessages] = useState([]);
const [inputText, setInputText] = useState('');
const [loading, setLoading] = useState(false);
const [error, setError] = useState('');
const handleSubmit = async () => {
if (!inputText.trim()) {
setError('Please enter a message.');
return;
}
setLoading(true);
setError('');
const newMessages = [...messages, { role: 'user', content: inputText }];
setMessages(newMessages);
setInputText('');
try {
const response = await fetch('/api/message', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ messages: newMessages })
});
const data = await response.json();
setMessages([...newMessages, { role: 'assistant', content: data.response }]);
} catch (e) {
setError('Failed to get response from server.');
} finally {
setLoading(false);
}
};
return (
<div className={styles.container}>
<Head>
<title>AI Chat with Next.js</title>
</Head>
<main className={styles.main}>
<h1 className={styles.title}>AI Chat with Next.js</h1>
<div className={styles.chatContainer}>
<div className={styles.chatWindow}>
{messages.map((msg, index) => (
<p key={index} className={msg.role}>{msg.content}</p>
))}
</div>
{error && <p className={styles.error}>{error}</p>}
<textarea
value={inputText}
onChange={(e) => setInputText(e.target.value)}
placeholder="Type your message here..."
/>
<button onClick={handleSubmit} disabled={loading}>
{loading ? 'Sending...' : 'Send'}
</button>
</div>
</main>
</div>
);
}
</code>
<p>For <b>streaming responses</b>, create <code>stream.js</code> in the <code>pages/api</code> directory:</p>
<code>
// pages/api/stream.js
import { Configuration, OpenAIApi } from 'openai';
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY
});
const openai = new OpenAIApi(configuration);
export default async function handler(req, res) {
res.setHeader('Content-Type', 'text/event-stream');
res.setHeader('Cache-Control', 'no-cache');
res.setHeader('Connection', 'keep-alive');
const { messages } = req.body;
try {
openai.createChatCompletion({
model: "gpt-3.5-turbo",
messages: [
{ role: "system", content: "You are an interactive chatbot." },
...messages
],
stream: true,
}).then(responseStream => {
responseStream.on('data', data => {
const decoded = new TextDecoder().decode(data.body);
const lines = decoded.trim().split('\n').filter(line => line);
for (const line of lines) {
if (line === '[DONE]') {
res.end();
} else {
const part = line.replace(/^data: /, '');
if (part) {
res.write(`data: ${part}\n\n`);
}
}
}
}).catch(error => {
res.write(`data: {"error": "Stream failed"}\n\n`);
res.end();
});
});
} catch (error) {
res.status(500).json({ error: 'Error generating stream from OpenAI' });
}
}
</code>
<p>Update the front-end to handle streaming responses:</p>
<code>
import { useState, useEffect } from 'react';
import Head from 'next/head';
import styles from '../styles/Home.module.css';
export default function Home() {
const [messages, setMessages] = useState([]);
const [inputText, setInputText] = useState('');
const [loading, setLoading] = useState(false);
const [error, setError] = useState('');
let eventSource;
const handleSubmit = () => {
if (!inputText.trim()) {
setError('Please enter a message.');
return;
}
setLoading(true);
setError('');
const newMessages = [...messages, { role: 'user', content: inputText }];
setMessages(newMessages);
setInputText('');
eventSource?.close();
eventSource = new EventSource('/api/stream');
eventSource.onmessage = function (event) {
try {
const data = JSON.parse(event.data);
const AIMessage = { role: 'assistant', content: data.response };
setMessages((prevMessages) => [...prevMessages, AIMessage]);
} catch (err) {
console.error(err);
setError('Error processing response from server.');
}
};
eventSource.onerror = function () {
setError('Error with server stream.');
setLoading(false);
eventSource?.close();
};
setLoading(false);
};
useEffect(() => {
return () => {
eventSource?.close();
};
}, []);
return (
<div className={styles.container}>
<Head>
<title>AI Chat with Next.js</title>
</Head>
<main className={styles.main}>
<h1 className={styles.title}>AI Chat with Next.js</h1>
<div className={styles.chatContainer}>
<div className={styles.chatWindow}>
{messages.map((msg, index) => (
<p key={index} className={msg.role}>{msg.content}</p>
))}
</div>
{error && <p className={styles.error}>{error}</p>}
<textarea
value={inputText}
onChange={(e) => setInputText(e.target.value)}
placeholder="Type your message here..."
/>
<button onClick={handleSubmit} disabled={loading}>
{loading ? 'Sending...' : 'Send'}
</button>
</div>
</main>
</div>
);
}
</code>
</section>
<section id="section-7">
<p>This setup integrates OpenAI's APIs into a Next.js application, providing real-time dynamic chat completions and handling streaming responses. These features enhance interactivity and user experience in your web application. By leveraging the power of AI, developers can create more engaging and responsive interfaces that can understand and respond to user inputs in natural language.</p>
<p>Some key benefits of this integration include:</p>
<ul>
<li><b>Real-time responses:</b> Users receive immediate feedback, improving engagement.</li>
<li><b>Scalability:</b> Next.js's server-side rendering capabilities ensure smooth performance even with complex AI interactions.</li>
<li><b>Customizability:</b> Developers can fine-tune the AI's responses and behavior to suit specific use cases.</li>
</ul>
<p>Remember to handle API rate limits and implement proper error handling to ensure a smooth user experience. Additionally, consider implementing features like <i>conversation history</i> and <i>context management</i> to make the AI interactions more coherent and context-aware.</p>
</section>
<section id="section-8">
<p><a href="https://www.writio.com">Revolutionize your content with Writio</a> - an AI writer that creates premium articles. This page was <a href="https://www.writio.com">crafted by Writio</a>.</p>
</section>
<section id="references">
<ol>
<li>OpenAI. GPT-3.5 Turbo. OpenAI API Documentation. 2023.</li>
<li>Vercel. Next.js Documentation. 2023.</li>
<li>Brown T, Mann B, Ryder N, et al. Language Models are Few-Shot Learners. Advances in Neural Information Processing Systems. 2020;33:1877-1901.</li>
</ol>
</section>
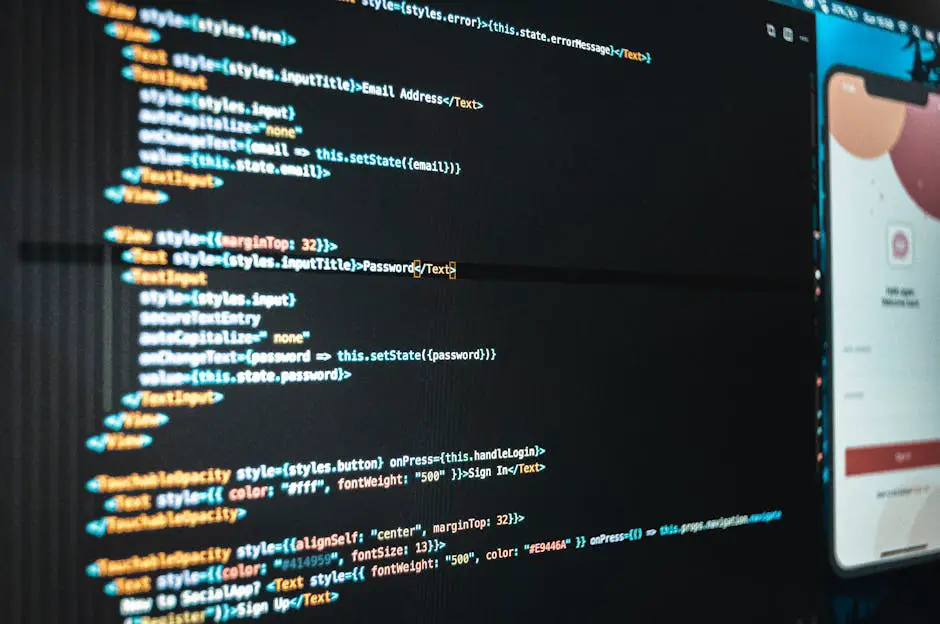
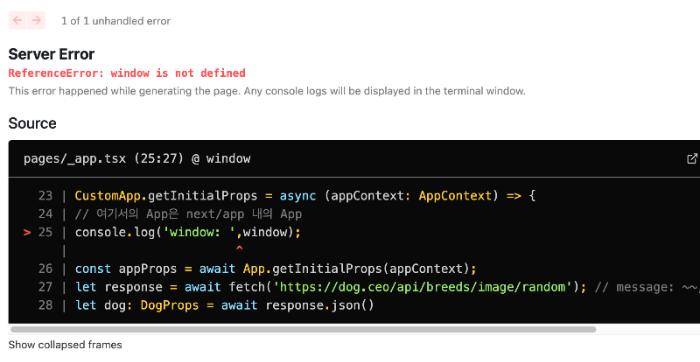