The Document Object Model (DOM) serves as the bridge between static HTML documents and the dynamic functionality provided by JavaScript. The DOM is essential for creating interactive, user-friendly websites that respond to user input and dynamically update content.
In this article, we delve into the DOM’s structure, explore its interaction with JavaScript, and provide practical examples to help you unlock its potential.
🌐 What Is the DOM?
The Document Object Model (DOM) is a programming interface for web documents. It represents the structure of an HTML or XML document as a tree of objects, allowing developers to manipulate content, structure, and styling using JavaScript.
Think of the DOM as a live map of your webpage, where each HTML element becomes a node that you can interact with.
🧩 How Does the DOM Work with JavaScript?
JavaScript uses the DOM to access and modify HTML elements dynamically. By using the DOM, developers can:
- Add, remove, or update elements and attributes.
- Respond to user actions like clicks or keystrokes.
- Style elements dynamically.
Here’s a simple example:
HTML Structure:
<!DOCTYPE html>
<html lang="en">
<head>
<title>DOM Example</title>
</head>
<body>
<h1 id="title">Hello, World!</h1>
<button id="changeButton">Change Text</button>
</body>
</html>
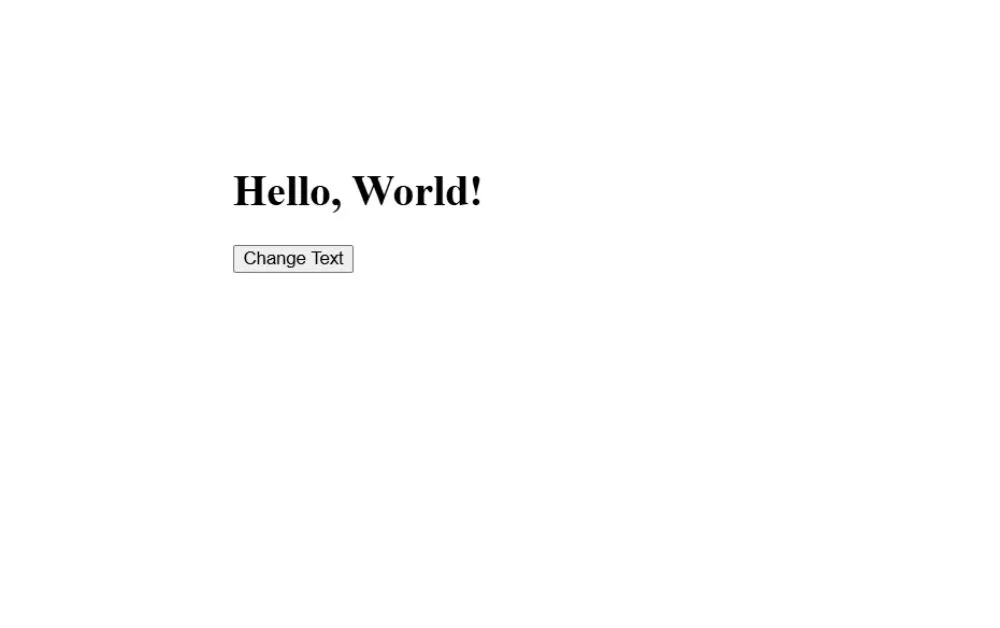
JavaScript Code:
// Select elements from the DOM
const title = document.getElementById('title');
const button = document.getElementById('changeButton');
// Add an event listener to the button
button.addEventListener('click', () => {
title.textContent = 'Text Changed!';
});
In this example, JavaScript dynamically updates the text of the <h1>
element when the button is clicked.
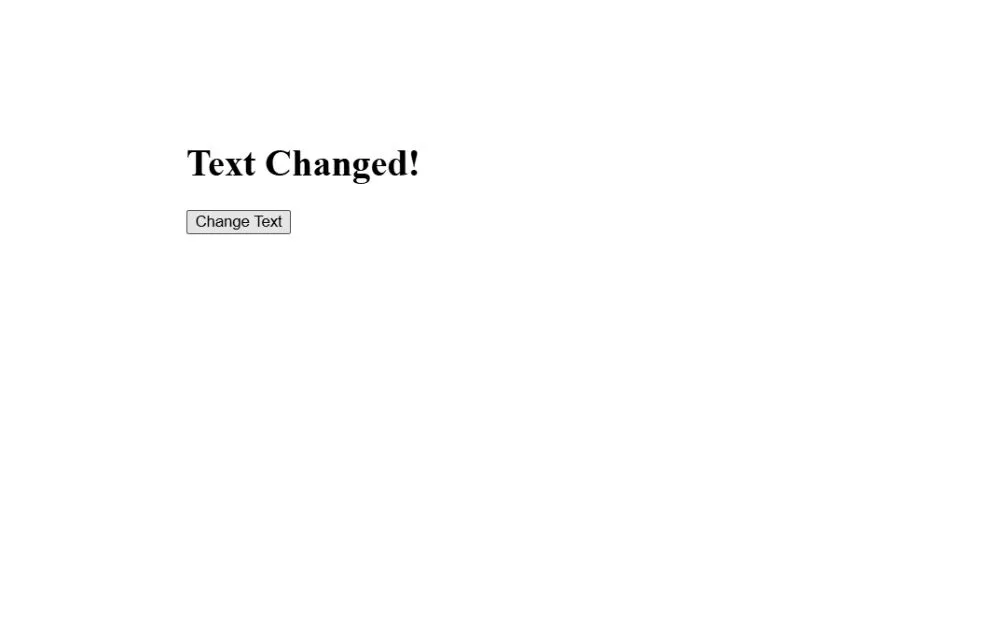
🔍 Key Components of the DOM
- Nodes: The DOM tree consists of nodes, each representing an element, attribute, or text.
- Document Object: The
document
object serves as the entry point for interacting with the DOM. - DOM Methods: Common methods include:
getElementById()
: Selects an element by its ID.querySelector()
: Selects the first element matching a CSS selector.createElement()
: Creates a new element.
- Events: The DOM enables interaction through events like
click
,keyup
, ormouseover
.
✨ Practical Applications of the DOM
The DOM powers dynamic features that make modern websites engaging. Here are a few examples:
1. Form Validation
const form = document.querySelector('form');
form.addEventListener('submit', (e) => {
const input = document.querySelector('#name');
if (!input.value) {
alert('Name is required!');
e.preventDefault();
}
});
2. Dynamic Content Loading
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
const container = document.querySelector('#dataContainer');
data.forEach(item => {
const div = document.createElement('div');
div.textContent = item.name;
container.appendChild(div);
});
});
⚡ DOM Traversal and Manipulation
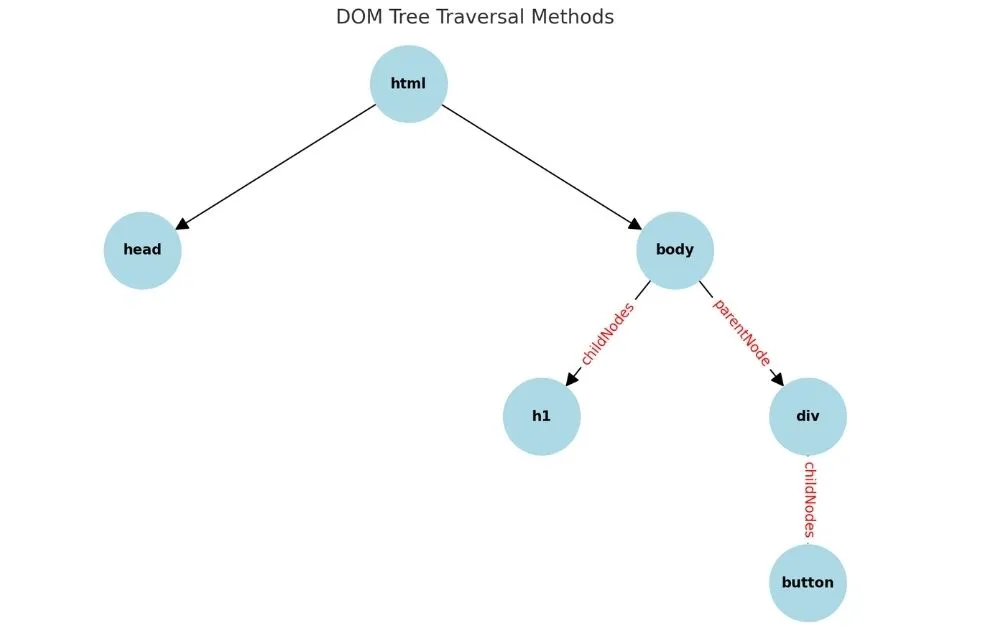
Navigating the DOM tree is fundamental for accessing and modifying specific elements.
Traversal Methods:
parentNode
: Access the parent of a node.childNodes
: Access all children of a node.nextSibling
andpreviousSibling
: Move between sibling nodes.
Example:
const list = document.querySelector('ul');
list.childNodes.forEach(node => {
console.log(node.textContent);
});
🛠️ Common DOM Methods and Properties
Method/Property | Description |
---|---|
document.getElementById | Selects an element by its ID. |
document.querySelector | Selects the first element matching a selector. |
element.innerHTML | Gets or sets the HTML content of an element. |
element.style | Modifies the style of an element. |
📝 Final Thoughts
Mastering the DOM is essential for building dynamic, interactive web applications. By understanding its structure and leveraging its methods, you can create websites that not only look great but also provide seamless user experiences.
Whether you’re updating content, responding to user actions, or building complex features, the DOM is your gateway to a truly interactive web.
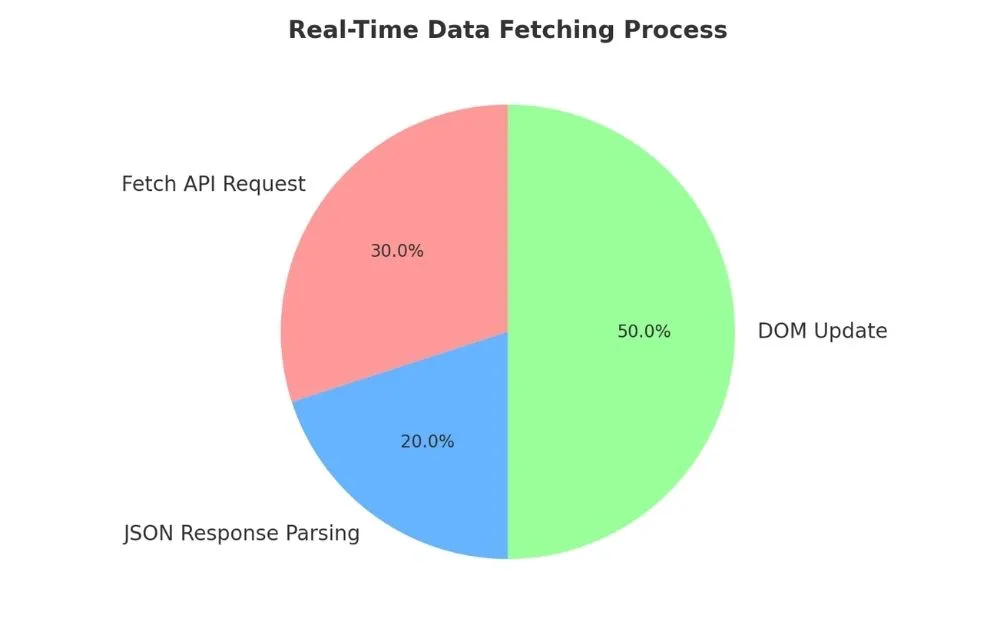
FAQs About DOM in JavaScript
- What is the DOM in simple terms?
The DOM is a structured representation of your webpage that JavaScript can manipulate. - Is the DOM part of JavaScript?
No, the DOM is a web standard. JavaScript interacts with it to create dynamic web pages. - Can the DOM be used with other programming languages?
Yes, many languages like Python (via frameworks) can interact with the DOM.
Understanding the HTML onclick Attribute