The @import
rule in CSS allows developers to import external stylesheets into their current stylesheet. This method of linking stylesheets provides a way to break up complex styling into manageable sections or share styles across multiple pages.
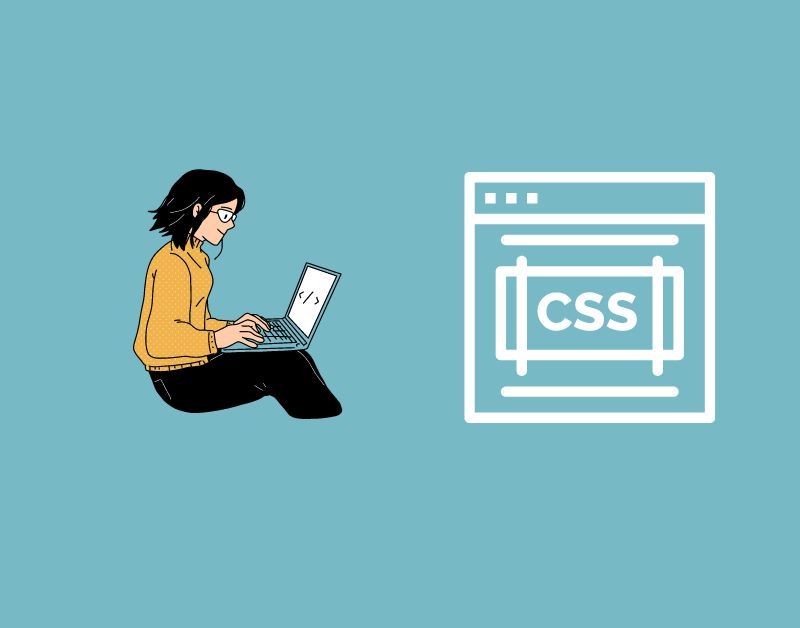
What is @import
?
The @import
rule is a CSS at-rule used to import styles from another stylesheet. This rule is typically placed at the top of a CSS file before any other declarations.
Syntax
@import url("styles.css");
The URL inside the parentheses points to the external stylesheet that you want to import. The URL can be an absolute or relative path, depending on where the stylesheet is located.
Basic Example
@import url("https://example.com/styles/main.css");
body {
font-family: Arial, sans-serif;
}
In this example, the @import
rule will load the styles from the external CSS file before applying the styles declared afterward, like setting the font-family
for the body.
Expanded @import
Syntax
CSS @import
has evolved, and now it supports more advanced features such as layering, media queries, and feature detection using supports()
. Here are some extended use cases:
1. Basic Import
@import url("styles.css");
This is the standard method of importing a stylesheet. The URL points to the CSS file you wish to import.
2. Import with Layer
@import url("styles.css") layer;
@import url("styles.css") layer(layer-name);
The layer
keyword allows you to assign the imported styles to a specific layer. Layers control the cascade order, which can help when managing larger stylesheets by grouping them logically.
layer
: Places the imported stylesheet in a default layer.layer(layer-name)
: Assigns the imported stylesheet to a named layer.
3. Import with supports()
@import url("styles.css") supports(display: grid);
@import url("styles.css") layer(layer-name) supports(display: grid);
The supports()
function ensures that the stylesheet is only imported if the browser supports certain CSS features. For example, you can import a file conditionally if display: grid
is supported by the browser.
4. Import with Media Queries
@import url("styles.css") screen and (max-width: 600px);
@import url("styles.css") layer(layer-name) list-of-media-queries;
You can also import stylesheets based on media queries, which makes it easier to serve different styles for different devices. Media queries are typically used to create responsive designs.
5. Full Syntax Example
@import url("styles.css") layer(layer-name) supports(display: grid) screen and (max-width: 600px);
This example imports a stylesheet into a specific layer, only if the display: grid
feature is supported, and only for screens smaller than 600 pixels wide.
Syntax Variations:
Here’s a breakdown of the variations:
- Basic URL Import:
@import url("styles.css");
- Import with Layer:
@import url("styles.css") layer;
@import url("styles.css") layer(layer-name);
- Import with
supports()
:
@import url("styles.css") supports(display: grid);
- Import with Layer and
supports()
:
@import url("styles.css") layer(layer-name) supports(display: grid);
- Import with Media Queries:
@import url("styles.css") screen and (max-width: 600px);
- Import with Layer,
supports()
, and Media Queries:
@import url("styles.css") layer(layer-name) supports(display: grid) screen and (max-width: 600px);
Benefits of Using @import
- Modularity: You can split large CSS files into smaller, more manageable chunks. For example, you can create separate stylesheets for layout, typography, and colors, and then import them into a master stylesheet.
- Reusability: Styles that are reused across multiple pages or sections can be stored in a single stylesheet and imported wherever needed.
- Maintainability: Changes made in a single imported file will be reflected across all pages where that file is included.
Best HTML Fonts: Top Choices for Your Website’s Typography
Media Queries with @import
You can use media queries to apply different styles based on conditions such as screen size or device type:
@import url("mobile.css") screen and (max-width: 600px);
In this example, mobile.css
will only be imported if the screen size is 600 pixels or less.
Browser Performance Considerations
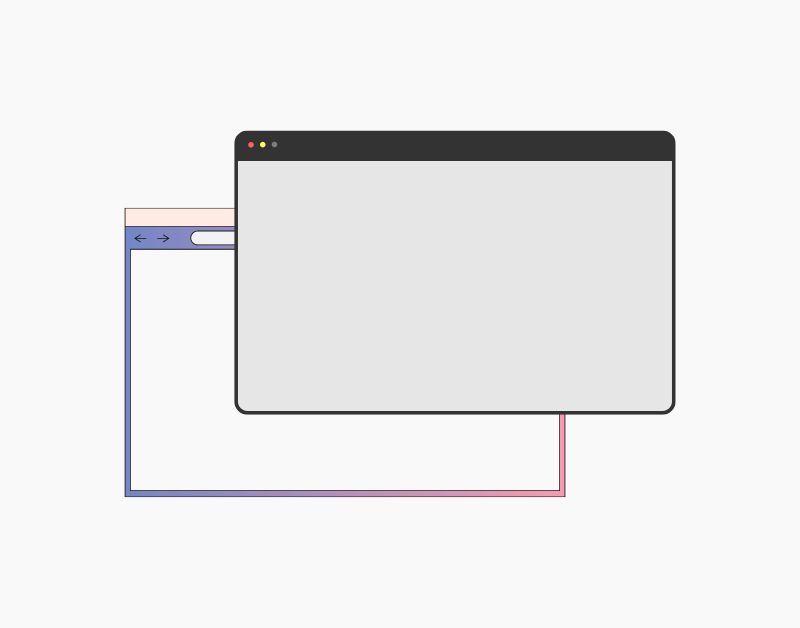
While @import
can be convenient, there are some performance concerns to keep in mind:
- Additional HTTP Requests: Each imported file creates an additional HTTP request, which can slow down page load times. Modern web development often prefers linking stylesheets directly in the HTML file using
<link>
tags to avoid extra requests. - Cascading Issues: Since styles are loaded in the order they are imported, it’s possible to unintentionally override styles if you aren’t careful about how you organize your imports.
HTTP Status Code: Understanding the Basics
Solution: Combining Stylesheets
One way to address performance concerns is by combining multiple stylesheets into one during the build process using tools like Webpack or Gulp. This minimizes the number of HTTP requests while still allowing developers to write modular CSS.
What Is Vite.js & Why It Is Better Than Webpack?
Difference Between @import
and <link>
The <link>
tag is an HTML element used to link external stylesheets, while @import
is a CSS rule. Though both achieve the same goal, they differ in how they behave.
@import
in CSS:
- Placement: Must be at the top of a CSS file.
- Load timing: Stylesheets are loaded only after the main stylesheet is loaded, potentially delaying rendering.
<link>
in HTML:
- Placement: Usually placed in the
<head>
section of an HTML file. - Load timing: Stylesheets are loaded as they are encountered, making them faster in some cases.
<!-- Using the link tag in HTML -->
<link rel="stylesheet" href="styles.css">
Best Practice
For better performance and quicker load times, it is generally recommended to use the <link>
tag in HTML rather than relying on @import
for external stylesheets. However, @import
can still be useful in modularizing your CSS, especially in specific use cases like media queries or layering.
Conclusion
The @import
rule in CSS is a helpful tool for keeping your styles organized and modular. However, due to its performance limitations, it’s best to use it sparingly in production environments. Instead, prefer using <link>
tags in your HTML, or combine multiple CSS files into one during your build process.
For further reference, you can explore the detailed documentation on MDN Web Docs.